Intercepting XHR (XMLHttpRequest) requests can be incredibly useful for debugging, monitoring network activity, or testing API calls in real-time. The following JavaScript snippet demonstrates how to override the XMLHttpRequest methods to log all request and response details directly to the console.
The Code
Here’s the snippet to intercept and log XHR requests and responses:
(function () {
const originalOpen = XMLHttpRequest.prototype.open;
const originalSend = XMLHttpRequest.prototype.send;
XMLHttpRequest.prototype.open = function (method, url, async, user, password) {
this._method = method;
this._url = url;
this._async = async;
this._user = user;
this._password = password;
console.log(`[XHR Intercepted] Opened: ${method} ${url}`);
return originalOpen.apply(this, arguments);
};
XMLHttpRequest.prototype.send = function (body) {
console.log(`[XHR Intercepted] Sending Request: ${this._method} ${this._url}`);
if (body) {
console.log(`[XHR Intercepted] Request Body:`, body);
}
this.addEventListener('readystatechange', function () {
if (this.readyState === 4) { // Ready state 4 means the request is complete
console.log(`[XHR Intercepted] Response for ${this._method} ${this._url}:`, this.responseText);
}
});
return originalSend.apply(this, arguments);
};
})();
How It Works
1. Override open Method:
The open method is intercepted to capture and log the HTTP method (GET, POST, etc.), the URL, and other optional parameters. These details are stored in custom properties (_method, _url, etc.) for later use.
2. Override send Method:
The send method is intercepted to log the request body (if any) and set up a listener for the readystatechange event.
3. Log Responses:
Once the request is complete (readyState === 4), the response is logged to the console along with the request details.
Use Cases
- Debugging: Easily monitor the flow of API requests and responses for troubleshooting.
- Testing APIs: Inspect request payloads and verify response structures without needing external tools.
- Learning: A great way for developers to understand how web applications communicate with back-end servers.
Precautions
- Performance Impact: While intercepting XHR requests, the logging might slow down the application slightly, especially in high-traffic environments.
- Read-Only: This snippet only logs requests and responses; it doesn’t modify them. Modifications would require additional logic.
How to Use
- Copy the code and paste it into the browser’s developer console or inject it into your page script.
- Make XHR requests as usual. The logs will appear in the console, showing details for each request and response.
Example:
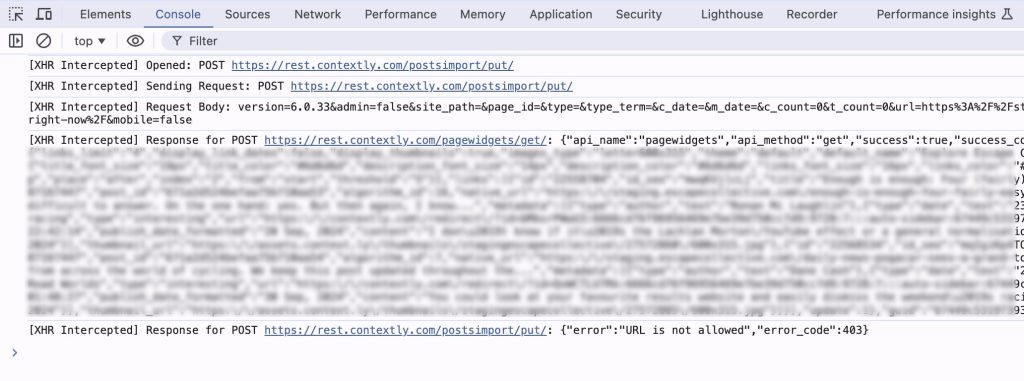
Leave a Reply
You must be logged in to post a comment.